Lensmaster allows you to output a real-time data feed of focus, iris, and zoom values to enable integration with custom FIZ motor setups. This guide will show you how to interface your custom FIZ setup with Lensmaster.
Prerequisites
- Lensmaster Beta 0.2.9 or newer
- Computer or Micro-Controller with networking capabilities
- Custom FIZ motor setup
In Lensmaster
- Navigate to Edit -> Preferences -> FIZ
- Under Lens Motor Configuration select “Camerabotics Custom FIZ”
- Enter the IP and port of the client you wish to send the data to.
- Enter the Data Rate (number of transmissions per second) you wish to use.
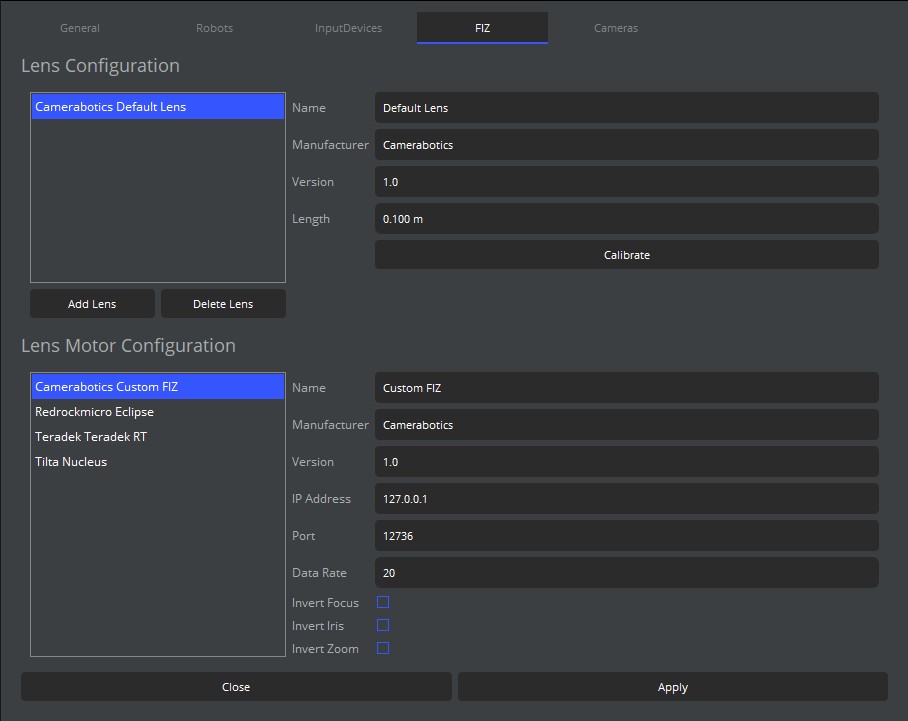
Output Data Format
Lensmaster outputs FIZ values over UDP in JSON format as shown below:
{
"timestamp_ns": 1612005091549420000,
"focus": 32767,
"iris": 32767,
"zoom": 32767
}
Focus, Iris, and Zoom values range from 0 to 65535. A timestamp in nano-seconds is also included indicating the time the data was transmitted.
Your custom FIZ control software is required to receive the transmitted data and translate Focus, Iris, and Zoom values to the motor’s rotation.
Client Code Samples
You can use the samples provided below as a starting point to develop your custom FIZ control client.
import json
import socket
# Create a UDP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.setsockopt(socket.SOL_SOCKET, socket.SO_RCVBUF, 1)
# Bind the socket to the port
server_address = ("", 12736)
print('starting up on %s port %s' % server_address)
sock.bind(server_address)
# Listen for incoming connections
while True:
# Wait for a connection
print('waiting for a connection')
try:
while True:
# Receive FIZ data from lensmaster
raw_data, addr = sock.recvfrom(1024)
data_str = raw_data.decode("UTF-8")
data = json.loads(data_str)
print(data['focus'])
except ConnectionError as e:
print(e)
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.nio.charset.StandardCharsets;
public class CustomFIZUDPClient {
public static void main(String[] args) throws IOException {
// Setup UDP socket
int port = 12736;
DatagramSocket socket = new DatagramSocket(port);
while (true) {
// Receive Data
byte[] buffer = new byte[1024];
DatagramPacket request = new DatagramPacket(buffer, buffer.length);
socket.receive(request);
// Deserialize Data
String json_str = new String(buffer, StandardCharsets.UTF_8).trim();
JsonParser parser = new JsonParser();
JsonElement jsonTree = parser.parse(json_str);
JsonObject jsonObject = jsonTree.getAsJsonObject();
int timestamp = (int) jsonObject.get("timestamp_ns").getAsInt();
int focus = (int) jsonObject.get("focus").getAsInt();
int iris = (int) jsonObject.get("iris").getAsInt();
int zoom = (int) jsonObject.get("zoom").getAsInt();
// Replace with motor control code
System.out.println(focus);
}
}
}